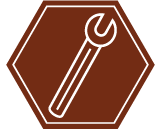
WordPress: Display All Post Attachment Images In A Slider
The Issue
There are a lot of scenarios I can think of where you may need to do something like this, but let’s say for this particular example you would like to make your client’s life easy by allowing them to upload images to a post, and then having those images automatically output into an image slider on their published page…no plugins, no shortcodes, just magic.
Note
This method assumes you are familiar with editing theme files and including the necessary scripts and markup to create sliders. I’m not going through all of the steps involved with this, just the method for grabbing all of the photos attached to a particular post.
The Solution
We can pull all of the post attachments with the following function (add this to your functions.php file):
function revconcept_get_images($post_id) { global $post; $thumbnail_ID = get_post_thumbnail_id(); $images = get_children( array('post_parent' => $post_id, 'post_status' => 'inherit', 'post_type' => 'attachment', 'post_mime_type' => 'image', 'order' => 'ASC', 'orderby' => 'menu_order ID') ); if ($images) : foreach ($images as $attachment_id => $image) : if ( $image->ID != $thumbnail_ID ) : $img_alt = get_post_meta($attachment_id, '_wp_attachment_image_alt', true); //alt if ($img_alt == '') : $img_alt = $image->post_title; endif; $big_array = image_downsize( $image->ID, 'large' ); $img_url = $big_array[0]; echo '<li>'; echo '<img src="'; echo $img_url; echo '" alt="'; echo $img_alt; echo '" />'; echo '</li><!--end slide-->'; endif; endforeach; endif; }
Note that this function DOES NOT include featured images attached to the post. If you want the featured image in the slider as well, see the alternate code in the example below.
This function pulls the images, with their alt attributes if they exist…if they don’t it will insert the post title as the alt attribute. It’s also setup to give you a choice in the size of the images that will be pulled, in this example it’s pulling the “large” image size, but you can change this to another image size, or even a custom image size that you’ve previously setup, just change “large” on this line: $big_array = image_downsize( $image->ID, 'large' );
The function outputs the images wrapped in <li>
tags. For this example I was using FlexSlider by WooThemes (but you can easily modify this to work with another image slider). The last step in making this work, is to add the following to your template file:
<div class="flexslider"> <ul class="slides"> <?php revconcept_get_images("$post->ID"); ?> </ul> </div><!--end flexslider-->
Again, note that some of this HTML markup is related to FlexSlider. The most important thing to include here is the <?php revconcept_get_images("$post->ID"); ?>
which calls your function.
(UPDATED) Include Featured Images
If you want to include the featured images attached to your page/post, use this code instead:
function revconcept_get_images($post_id) { global $post; $thumbnail_ID = get_post_thumbnail_id(); $images = get_children( array('post_parent' => $post_id, 'post_status' => 'inherit', 'post_type' => 'attachment', 'post_mime_type' => 'image', 'order' => 'ASC', 'orderby' => 'menu_order ID') ); if ($images) : foreach ($images as $attachment_id => $image) : $img_alt = get_post_meta($attachment_id, '_wp_attachment_image_alt', true); //alt if ($img_alt == '') : $img_alt = $image->post_title; endif; $big_array = image_downsize( $image->ID, 'large' ); $img_url = $big_array[0]; echo '<li>'; echo '<img src="'; echo $img_url; echo '" alt="'; echo $img_alt; echo '" />'; echo '</li><!--end slide-->'; endforeach; endif; }
Conclusion
That’s it! This is a really simple way to make creating sliders super easy with minimal steps for your clients (or yourself, I use this method in my portfolio on this site).
Hi there!
This example works just fine, except one thing: In my case, it seems to only find one image, although I have connected three with this post. What could be the problem?
Thanks!
Hmmm…not sure what would be causing this. Try defining the post ID. Add this line to the top of the function:
Never mind!
I did something wrong and didn’t connect the pictures properly. All works fine, you are a live-saver!
All the best
Sebastian
I have found another issue, maybe you can help me here?
I realised, that your function finds all attached pictures of a post, except the one that is the “featured image”. Is there a way, to include that image also?
Sure, so just change this line
to this…
Perfect! Thanks!
Hello Guys,
In my case I combined this with a custom slider so that it is a horizontal slider with a scrollbar. my issue is that if i add the images it shows perfect when I remove them, they some how stay there does not matter if i delete everything they simply stay. any ideas?
Do you have any other photos on your WordPress site? Or are the ones you added to the post the only ones on the entire site? It sounds like you may be having this problem. You can read my reply for the fix ;)
Hello Chris.
I Got it working. it was not on the site that was on my link.
so all is good now. :) thanks in anycase.
Hi Chris,
This is exactly what I was looking for. I’m using MetaSlider (who uses flexslider), and it just works.
But there is one little thing that doesn’t work: I get a slider that shows all (!) attached images, regardless of the post you’re on.
This means I ended up with a slider with almost 50 images. The post I use has only 3 images attached.
So somewhere down the line there must be something going wrong.
I’m using it on a portfolio page, and I include the slider with a shortcode:
[metaslider id=601]
This is an empty slider.
I changed a bit on the part that has to be included in the template file (in my case this is ‘single-portfolio.php’):
add_action( ‘genesis_before_loop’, ‘addslider’);
function addslider() {
echo ”;
echo ”;
revconcept_get_images(“$post->ID”);
echo ”;
echo ”;
}
As you can see I added an extra ‘id=”metaslider_601″‘ to properly allocate the empty slider.
It all works, but I end up with a slider containing over 50 images, and not the ones attached to this specific post-id.
Do you know where I mess up things?
Hi Toon,
Someone has a similar problem with this and it was discussed in earlier comments on this post…let me try to find that and get back to you with a possible solution.
I found it….the issue was the $post_id variable. It may need to be defined, you can test to see what it’s outputting, or set it with:
Hello, is there a way to add the slider controls to it? Thanks
If you are using Flexslider, you can add/remove controls and features by adding some lines of code to the JavaScript that initializes the slider. You can find everything you need here:
http://www.woothemes.com/flexslider/
Hello Chris,
This is a great code you shared. It is helping me a lot. but I am having problems when I use the code a child theme. I am unable to see the slideshow working. It works in the parent theme but not in a child theme.
• I tried leaving the code in the parent theme’s functions.php
• I tried putting the code in the child theme’s functions.php
• I tried putting the code in both :p
Thanks in advance
The code in the first box in my example should go in your child theme functions.php.
The code in box two should go in a template file, like page.php or wherever you want the slider to show.
The code at the link I shared with you at Flexslider should be added like this:
1. Save this file (jquery.flexslider.js) into a folder in your child theme…probably called scripts or js, it doesn’t really matter.
2. Save this file (flexslider.css) into a folder in your child theme…probably called styles or css…again it doesn’t really matter.
3. Add these lines of code to your theme’s script file….if you don’t have one you can create one and just call is script.js:
Now, you need to tell your theme to use these files. The proper way to do this is to “enqueue” them in your functions.php file. Here is a link on how to do that:
http://www.wpbeginner.com/wp-tutorials/how-to-properly-add-javascripts-and-styles-in-wordpress/
You will know whether or not this is working if you “view source” on your website and you can see the files being called in the header and/or footer of the site. If you don’t see links to these files, you didn’t enqueue them properly.
Amazing!
I’ve made some ajusts to my needs and it works perfectly!
Excellent!