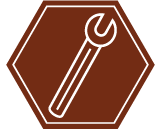
WordPress: Display All Post Attachment Images In A Slider
The Issue
There are a lot of scenarios I can think of where you may need to do something like this, but let’s say for this particular example you would like to make your client’s life easy by allowing them to upload images to a post, and then having those images automatically output into an image slider on their published page…no plugins, no shortcodes, just magic.
Note
This method assumes you are familiar with editing theme files and including the necessary scripts and markup to create sliders. I’m not going through all of the steps involved with this, just the method for grabbing all of the photos attached to a particular post.
The Solution
We can pull all of the post attachments with the following function (add this to your functions.php file):
function revconcept_get_images($post_id) { global $post; $thumbnail_ID = get_post_thumbnail_id(); $images = get_children( array('post_parent' => $post_id, 'post_status' => 'inherit', 'post_type' => 'attachment', 'post_mime_type' => 'image', 'order' => 'ASC', 'orderby' => 'menu_order ID') ); if ($images) : foreach ($images as $attachment_id => $image) : if ( $image->ID != $thumbnail_ID ) : $img_alt = get_post_meta($attachment_id, '_wp_attachment_image_alt', true); //alt if ($img_alt == '') : $img_alt = $image->post_title; endif; $big_array = image_downsize( $image->ID, 'large' ); $img_url = $big_array[0]; echo '<li>'; echo '<img src="'; echo $img_url; echo '" alt="'; echo $img_alt; echo '" />'; echo '</li><!--end slide-->'; endif; endforeach; endif; }
Note that this function DOES NOT include featured images attached to the post. If you want the featured image in the slider as well, see the alternate code in the example below.
This function pulls the images, with their alt attributes if they exist…if they don’t it will insert the post title as the alt attribute. It’s also setup to give you a choice in the size of the images that will be pulled, in this example it’s pulling the “large” image size, but you can change this to another image size, or even a custom image size that you’ve previously setup, just change “large” on this line: $big_array = image_downsize( $image->ID, 'large' );
The function outputs the images wrapped in <li>
tags. For this example I was using FlexSlider by WooThemes (but you can easily modify this to work with another image slider). The last step in making this work, is to add the following to your template file:
<div class="flexslider"> <ul class="slides"> <?php revconcept_get_images("$post->ID"); ?> </ul> </div><!--end flexslider-->
Again, note that some of this HTML markup is related to FlexSlider. The most important thing to include here is the <?php revconcept_get_images("$post->ID"); ?>
which calls your function.
(UPDATED) Include Featured Images
If you want to include the featured images attached to your page/post, use this code instead:
function revconcept_get_images($post_id) { global $post; $thumbnail_ID = get_post_thumbnail_id(); $images = get_children( array('post_parent' => $post_id, 'post_status' => 'inherit', 'post_type' => 'attachment', 'post_mime_type' => 'image', 'order' => 'ASC', 'orderby' => 'menu_order ID') ); if ($images) : foreach ($images as $attachment_id => $image) : $img_alt = get_post_meta($attachment_id, '_wp_attachment_image_alt', true); //alt if ($img_alt == '') : $img_alt = $image->post_title; endif; $big_array = image_downsize( $image->ID, 'large' ); $img_url = $big_array[0]; echo '<li>'; echo '<img src="'; echo $img_url; echo '" alt="'; echo $img_alt; echo '" />'; echo '</li><!--end slide-->'; endforeach; endif; }
Conclusion
That’s it! This is a really simple way to make creating sliders super easy with minimal steps for your clients (or yourself, I use this method in my portfolio on this site).
Thank you a lot! I’m not a pro and was looking for a decision for several hours. Now it works. Thank you a lot again!