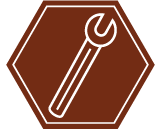
WordPress: Display All Post Attachment Images In A Slider
The Issue
There are a lot of scenarios I can think of where you may need to do something like this, but let’s say for this particular example you would like to make your client’s life easy by allowing them to upload images to a post, and then having those images automatically output into an image slider on their published page…no plugins, no shortcodes, just magic.
Note
This method assumes you are familiar with editing theme files and including the necessary scripts and markup to create sliders. I’m not going through all of the steps involved with this, just the method for grabbing all of the photos attached to a particular post.
The Solution
We can pull all of the post attachments with the following function (add this to your functions.php file):
function revconcept_get_images($post_id) { global $post; $thumbnail_ID = get_post_thumbnail_id(); $images = get_children( array('post_parent' => $post_id, 'post_status' => 'inherit', 'post_type' => 'attachment', 'post_mime_type' => 'image', 'order' => 'ASC', 'orderby' => 'menu_order ID') ); if ($images) : foreach ($images as $attachment_id => $image) : if ( $image->ID != $thumbnail_ID ) : $img_alt = get_post_meta($attachment_id, '_wp_attachment_image_alt', true); //alt if ($img_alt == '') : $img_alt = $image->post_title; endif; $big_array = image_downsize( $image->ID, 'large' ); $img_url = $big_array[0]; echo '<li>'; echo '<img src="'; echo $img_url; echo '" alt="'; echo $img_alt; echo '" />'; echo '</li><!--end slide-->'; endif; endforeach; endif; }
Note that this function DOES NOT include featured images attached to the post. If you want the featured image in the slider as well, see the alternate code in the example below.
This function pulls the images, with their alt attributes if they exist…if they don’t it will insert the post title as the alt attribute. It’s also setup to give you a choice in the size of the images that will be pulled, in this example it’s pulling the “large” image size, but you can change this to another image size, or even a custom image size that you’ve previously setup, just change “large” on this line: $big_array = image_downsize( $image->ID, 'large' );
The function outputs the images wrapped in <li>
tags. For this example I was using FlexSlider by WooThemes (but you can easily modify this to work with another image slider). The last step in making this work, is to add the following to your template file:
<div class="flexslider"> <ul class="slides"> <?php revconcept_get_images("$post->ID"); ?> </ul> </div><!--end flexslider-->
Again, note that some of this HTML markup is related to FlexSlider. The most important thing to include here is the <?php revconcept_get_images("$post->ID"); ?>
which calls your function.
(UPDATED) Include Featured Images
If you want to include the featured images attached to your page/post, use this code instead:
function revconcept_get_images($post_id) { global $post; $thumbnail_ID = get_post_thumbnail_id(); $images = get_children( array('post_parent' => $post_id, 'post_status' => 'inherit', 'post_type' => 'attachment', 'post_mime_type' => 'image', 'order' => 'ASC', 'orderby' => 'menu_order ID') ); if ($images) : foreach ($images as $attachment_id => $image) : $img_alt = get_post_meta($attachment_id, '_wp_attachment_image_alt', true); //alt if ($img_alt == '') : $img_alt = $image->post_title; endif; $big_array = image_downsize( $image->ID, 'large' ); $img_url = $big_array[0]; echo '<li>'; echo '<img src="'; echo $img_url; echo '" alt="'; echo $img_alt; echo '" />'; echo '</li><!--end slide-->'; endforeach; endif; }
Conclusion
That’s it! This is a really simple way to make creating sliders super easy with minimal steps for your clients (or yourself, I use this method in my portfolio on this site).
Lovely script, very useful. Thank you
Q. Do you know if there is a way to get the flexslider navigation buttons and arrows working with this as mine done seem to be working?
Hey Ben, do you have a link to where you are trying to use this? My immediate thought would be that you need to call those options in your Javascript. So, where you have something like this:
$('.flexslider').flexslider();
You need to make sure you add the following:
$('.flexslider').flexslider({
controlNav: true,
directionNav: true,
prevText: "Previous",
nextText: "Next",
});
sorry for late reply, worked it out, it was me being dumb. the laptop i was testing on happedend to be a touch screen. apparantely flexslider works this out and disables the controls for touch screens. !? so all is well, thanks again for your help, Ben
Hi, great job! is perfect :)
but I have a question …
this code shows “all” the images in the post … but if I want to show only the images attached to the post?
I need this for a shopping cart and unfortunately this code displays all pictures of the entire site. : S
thank you very much!
i’m from Chile
sorry! i have a mistake ;)
the code is perfect! :)
Glad it worked for you. Yes, this code should only show the photos attached to the post.
Hi Pablo, I’m trying asking you because you seemed having had the same problem I’m having now. I can see all media images (of the entire site) attached to my post, or any post or page I create from scratch without attaching anything. What did you find having mistaken? Many thanks!
Oops. It seems I replied to your comment in a new post… I really need to take classes! :-)
Haha…not a problem. You’re already putting in the effort to learn…you’ll get there, I have full confidence!
Thanks Chris! I had a look at the tutorials but maybe I haven’t dug deep enough. I’ll check there again.
….and by the way, congrats on your work which I find really inspiring!
Thank you so much!
Thanks a lot for this tutorial. I’m gonna ask a dumb question but I’m a newbie: I’m using the plugin slider pro and I don’t know which class I have to insert in your code instead of flexslider… Thanks in advance!
No such thing as a dumb question ;) This tutorial was written for sliders that are going to be built into a theme…not so much for plugins. Changing the class won’t get this to work for you. I haven’t personally used “Slider Pro”, but it works like most slider plugins. You should be able to choose images and add them to a slider, then go to a post/page and choose that slider from a dropdown.
Automatically pulling post attachments into “Slider Pro” probably isn’t one of their features.
Have you taken a look at this page? It has some video tutorials: http://codecanyon.net/item/slider-pro-wordpress-premium-slider-plugin/full_screen_preview/253501